To start the server java -jar selenium-server-standalone-2.48.2.jar
When you start the server, chrome and firefox will work
Because the official documentation says
Client mode: where the language bindings connect to the remote instance. This is the way that the FirefoxDriver, OperaDriver and the RemoteWebDriver client normally work.
Server mode: where the language bindings are responsible for setting up the server, which the driver running in the browser can connect to. The ChromeDriver works in this way.
For internet Explorer and Chrome where language bindings are responsible for setting up the server start the server like
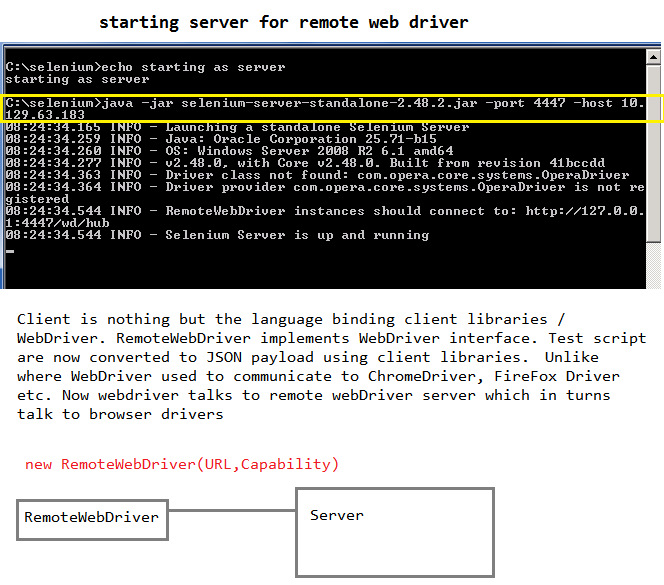
For Internet Explorer
C:\selenium>java -jar selenium-server-standalone-2.48.2.jar -Dwebdriver.ie.driver="C:\selenium\IEDriverServer_Win32_2.48.0\IEDriverServer.exe"
For Chrome
C:\selenium>java -jar selenium-server-standalone-2.48.2.jar -Dwebdriver.chrome.driver="C:\selenium\<path to chrome driver>
When you want to use multiple instance of same browser or multiple browser on same remote machine use GRID
Working with Internet Explorer
_____________________________
Start the remote server using the following command
java -jar selenium-server-standalone-2.48.2.jar -Dwebdriver.ie.driver="C:\selenium\IEDriverServer_Win32_2.48.0\IEDriverServer.exe"
Below code will open ie browser at remote location 10.129.63.183:4444
desiredCapabilities.setCapability(InternetExplorerDriver.INTRODUCE_FLAKINESS_BY_IGNORING_SECURITY_DOMAINS, true);
is used to ignoreProtectedModeSettings for several zones in IE.
public static void ieRemoteWebDriver(RemoteWebDriver rwd) {
DesiredCapabilities desiredCapabilities = DesiredCapabilities.internetExplorer();
desiredCapabilities.setCapability(InternetExplorerDriver.INTRODUCE_FLAKINESS_BY_IGNORING_SECURITY_DOMAINS, true);
try {
rwd = new RemoteWebDriver(new URL("http://10.129.63.183:4444/wd/hub"), desiredCapabilities);
} catch (MalformedURLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
Below code will open ie browser at remote location 10.129.63.183:4444
and will print browser details
public static void ieRemoteWebDriver(RemoteWebDriver rwd) {
DesiredCapabilities desiredCapabilities = DesiredCapabilities.internetExplorer();
desiredCapabilities.setCapability(InternetExplorerDriver.INTRODUCE_FLAKINESS_BY_IGNORING_SECURITY_DOMAINS,
true);
try {
rwd = new RemoteWebDriver(new URL("http://10.129.63.183:4444/wd/hub"), desiredCapabilities);
Capabilities actualCapabilityes = rwd.getCapabilities();
System.out.println(actualCapabilityes.getVersion());
System.out.println(actualCapabilityes.getBrowserName());
System.out.println(actualCapabilityes.getPlatform());
} catch (MalformedURLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
For setting Capability for browser selection . Common for all browsers
Key | Type | Description |
browserName | string | The name of the browser being used; should be one of {android|chrome|firefox|htmlunit|internet explorer|iPhone|iPad|opera|safari}. |
version | string | The browser version, or the empty string if unknown. |
platform | string | A key specifying which platform the browser should be running on. This value should be one of {WINDOWS|XP|VISTA|MAC|LINUX|UNIX|ANDROID}. When requesting a new session, the client may specify ANY to indicate any available platform may be used. For more information see [GridPlatforms] |
Setting desired capability for browser name
DesiredCapabilities desiredCapabilities = new DesiredCapabilities();
desiredCapabilities.setBrowserName("internet explorer");
desiredCapabilities.setCapability(InternetExplorerDriver.INTRODUCE_FLAKINESS_BY_IGNORING_SECURITY_DOMAINS,
true);
OR
DesiredCapabilities desiredCapabilities = DesiredCapabilities.internetExplorer();
desiredCapabilities.setCapability(InternetExplorerDriver.INTRODUCE_FLAKINESS_BY_IGNORING_SECURITY_DOMAINS,
true);
Setting desired capability for Version, Platform (example)
desiredCapabilities.setCapability("platform", Platform.ANY);
desiredCapabilities.setCapability("version", "11");
Working with Chrome Driver
_____________________________
desiredCapabilities.setCapability("platform", Platform.ANY);
desiredCapabilities.setCapability("version", "11");
- For a list of capability specific to Internet Explorer please refer HERE
Working with Chrome Driver
_____________________________
Start the server in remote machine
java -jar selenium-server-standalone-2.48.2.jar -Dwebdriver.chrome.driver="C:\selenium\chromedriver_win32\chromedriver.exe"
Hi Subhra Das,
ReplyDeleteThanks for the post. Very easily understandable, the testing capability you mentioned here seems informative one. I also request your viewers to also view this page about http://www.credosystemz.com/training-in-chennai/best-selenium-training-in-chennai/
Amazing, thanks a lot my friend, I was also siting like a your banner image when I was thrown into Selenium. When I started learning then I understood it has got really cool stuff.
ReplyDeleteI can vouch web driver has proved the best feature in Selenium framework.
Thanks a lot for taking a time to share a wonderful article.